My “Simply AWS” series is aimed at absolute beginners to quickly get started with the wonderful world of AWS, this one is about DynamoDB.
What is DynamoDB?
DynamoDB is a NoSQL, fully managed, key-value database within AWS.
Why should I use it?
When it comes to data storage, selecting what technology to use is always a big decisions, DynamoDB is like any other technology not a silver bullet but it does offer a lot of positives if you need a document based key-value storage.
Benefits of DynamoDB
- Low latency
- Scaleable and fully managed
- Cheap
- Highly durable
- Easy for beginners
- And much more
How do I start?
First you’re gonna need an AWS account, follow this guide.
Setting up our first DynamoDB database
If you feel like it you can set your region on the top right corner of the AWS console, it should default to us-east-1 but you can select something closer to you, read more about regions here.
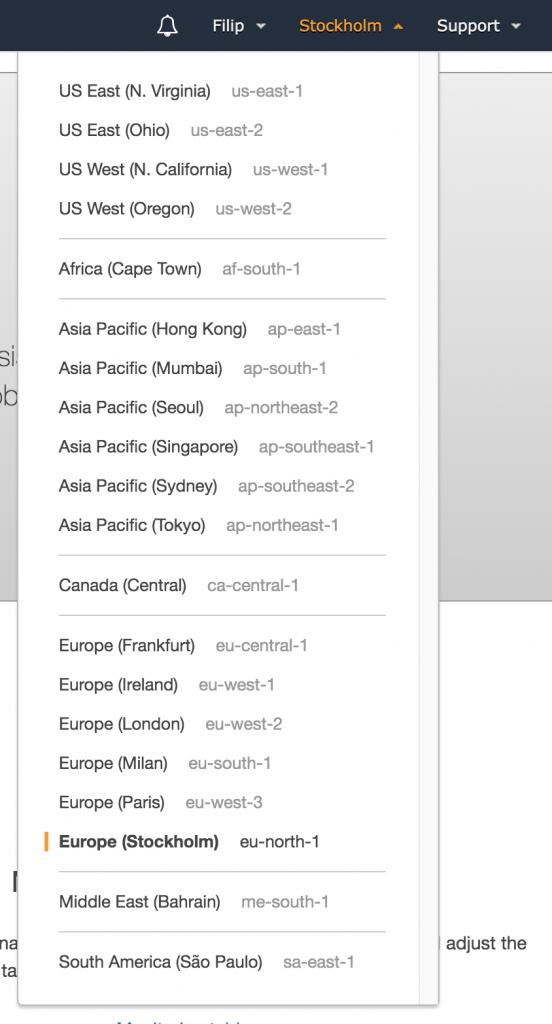
From the AWS console, head to Services and search for DynamoDB, select the first option.
The first time you open up DynamoDB you should see a blue button with the text Create Table, click it.
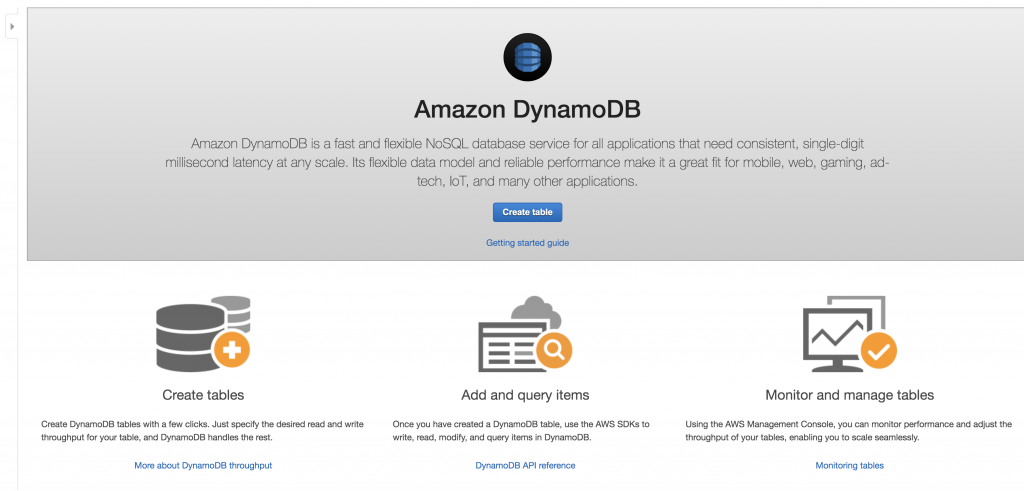
Now you’re presented with some options for creating your table, enter myFirstTable (this can be anything) in the Table name.
Primary key
A key in a database is something used to identify items in the table and as such it must always be unique for every item. In DynamoDB the key is built up by a Partion key and an option Sort key
- Partition Key: As the tooltip in the AWS console describes the Partion key is used to partion data across hosts because of that for best practice you should use an attribute that has a wide range of values, for now we don’t need to worry much about this, the main thing to takeaway is that if the Partion key is used alone it must be unique
- Sort key: if the optional sort key is included the partion key does not have to be unique (but the combination of partion key and sort key does) it allows us to sort within a partion.
Let’s continue, for this example I’m gonna say i’m creating something like a library system, so I’ll put Author as the Partion key and BookTitle as the sort Key.
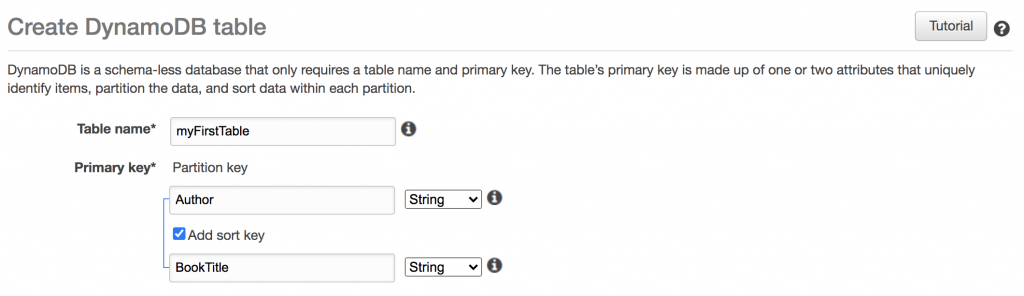
Note that this is just one of many ways you could setup this type of table and choosing a good primary key is arguably one of the most important decisions when creating a DynamoDB table, what’s good about AWS is that we can create a table, try it out, change our minds and just create a new one with ease.
Next up are table settings, these are things like secondary indexes, provisioned capacity, autoscaling, encryption and such. It’s a good idea to eventually get a bit comfortable with these options and I highly recommend looking into on-demand read/write capacity mode, but as we just want to get going now the default settings are fine and will not cost you anything for what we are doing today.
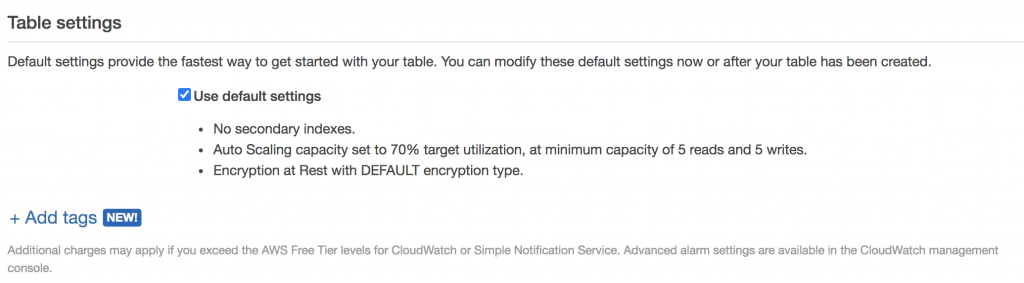
Hit create and wait for your table to be created!
Now you should be taken to the tables view of DynamoDB and your newly created table should be selected, this can be a bit daunting as there is a lot of options and information, but let’s head over to the Items tab.
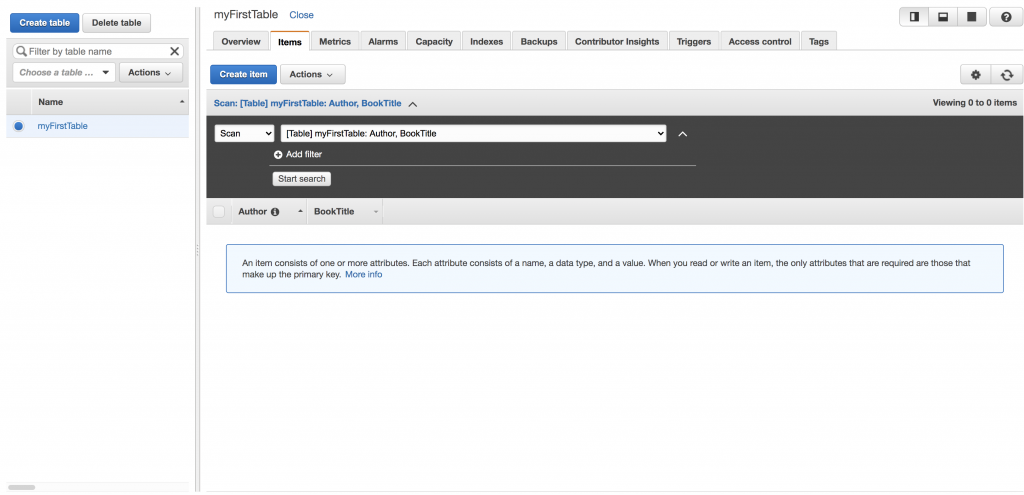
From here we could create an Item directly from the console (feel free to try it out if you want) but I think we can do one better and setup a lambda for interacting with the table.
Creating our first item
If you’ve never created an AWS lambda before I have written a similar guide to this one on the topic, you can find it here.
Create a lambda called DynamoDBInteracter
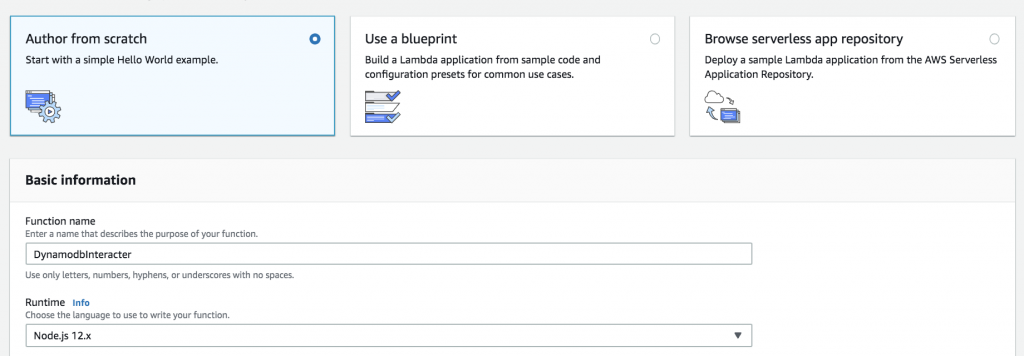
Make sure to select to create a new role from a template and search for the template role Simple microservice permissions (this will allow us to perform any actions agains DynamoDB).
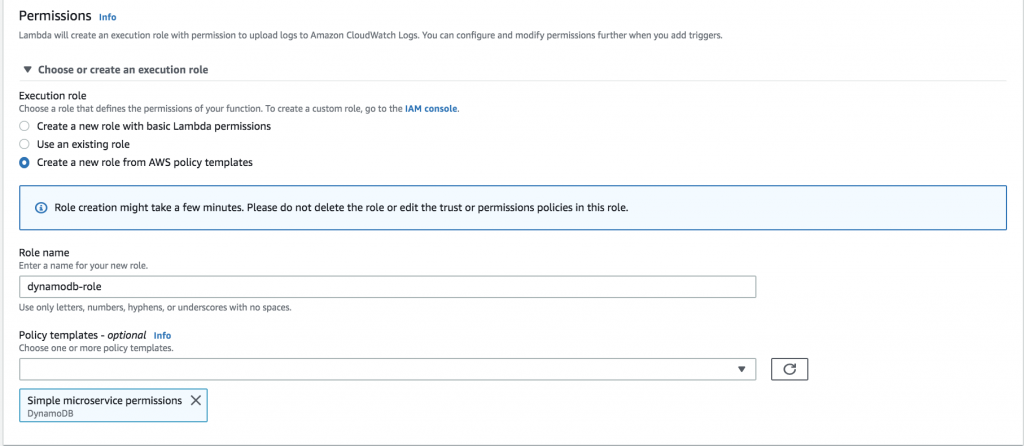
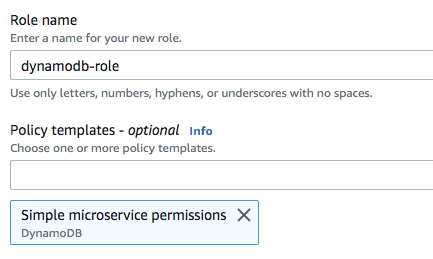
After creating the lambda we can directly edit it in the AWS console, copy and paste this code.
const AWS = require('aws-sdk')
const client = new AWS.DynamoDB.DocumentClient();
exports.handler = async (event) => {
try {
console.log(event.action)
console.log(event.options)
let response;
switch (event.action) {
case 'put':
response = await putItem(event.options);
break;
case 'get':
response = await getItem(event.options);
break;
}
return response;
} catch (e) {
console.error(e)
return e.message || e
}
};
let putItem = async (options) => {
var params = {
TableName : 'myFirstTable',
Item: {
Author: options.author,
BookTitle: options.bookTitle,
genre: options.genre
}
};
return await client.put(params).promise();
}
let getItem = async (options) => {
var params = {
TableName : 'myFirstTable',
Key: {
Author: options.author,
BookTitle: options.bookTitle
}
};
return await client.get(params).promise();
}
hit Save then create a new test event like this.
{
"action": "put",
"options": {
"author": "Douglas Adams",
"bookTitle": "The Hitchhiker's Guide to the Galaxy",
"genre": "Sci-fi"
}
}
and run that test event.
Go back to DynamoDB and the Items tab and you should see your newly created Item!
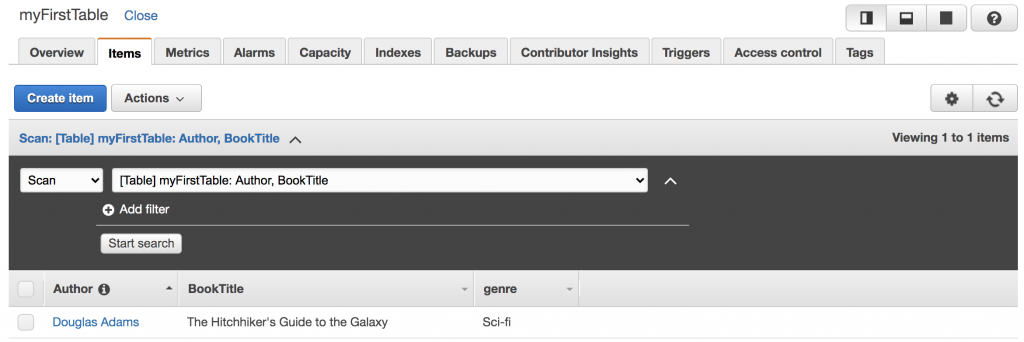
Notice that we did not have to specify the genre attribute that is because DynamoDB is NoSQL it follows no schemea and any field + value can be added to any item irregardless of the other items composition as long as the primary key is valid.
Retrieving our item
Now let’s try to get that item, create another test event like this.
{
"action": "get",
"options": {
"author": "Douglas Adams",
"bookTitle": "The Hitchhiker's Guide to the Galaxy"
}
}
and run it.
You can expand the execution results and you should see your response with the full data of the item.
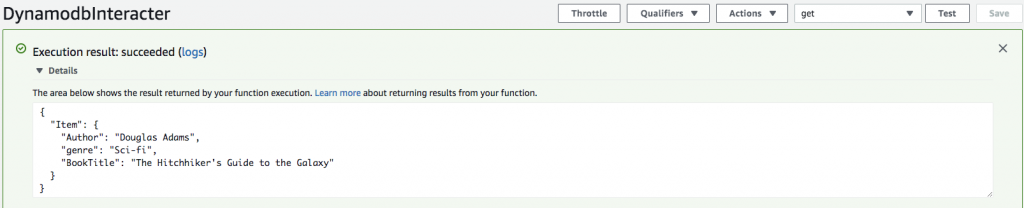
Congratulations!
You’ve just configured your first DynamoDB table and performed calls against it with a lambda but don’t stop here the possibilities with just these two AWS services are endless, my next guide in this series will cover API-Gateway and how we can connect an API to our lambda that then communicates with our database table, stay tuned!
What’s next?
As I’m sure you understand we’ve just begun to scratch the surface of what DynamoDB has to offer, my goal with this series of guides is to get your foot in the door with some AWS services and show that although powerful and vast they are still easy to get started with, to check out more of what calls can be made with the DynamoDB API (such as more complicated queries, updates and scans as well as batch writes and reads) check this out, feel free to edit the code and play around.
I would also like to recommend this guide if you want even more in-depth look into DynamoDB, it covers most of what I have here but more detailed and also goes over some of the API actions mentioned above.
Contact me
Questions? Thoughts? Feedback?
Twitter: @tqfipe
Linkedin: Filip Pettersson